4.2.2 mapping
Mappings are are really just more generic arrays. However, they are slower
and use more memory than arrays, so they cannot replace arrays completely.
What makes mappings special is that they can be indexed on other things than
integers. We can imagine that a mapping looks like this:
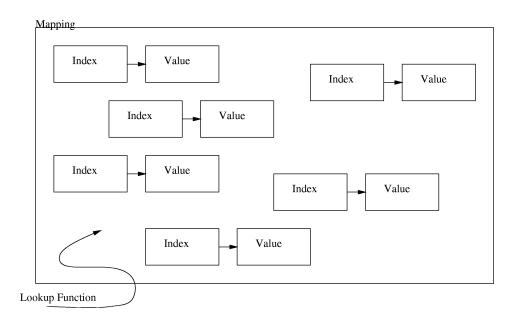
fig 4.2
Each index-value pair is floating around freely inside the mapping. There is
exactly one value for each index. We also have a (magical) lookup function.
This lookup function can find any index in the mapping very quickly. Now, if
the mapping is called m and we index it like this:
m [ i ] the lookup function will quickly find the index
i in the mapping and return the corresponding value. If the index is
not found, zero is returned instead.
If we on the other hand assign an index in the mapping the value will
instead be overwritten with the new value. If the index is not found when
assigning, a new index-value pair will be added to the mapping.
Writing a constant mapping is easy:
([ ]) // Empty mapping
([ 1:2 ]) // Mapping with one index-value pair, the 1 is the index
([ "one":1, "two":2 ]) // Mapping which maps words to numbers
([ 1:({2.0}), "":([]), ]) // Mapping with lots of different types
As with arrays, mappings can contain any type. The main difference is that
the index can be any type too. Also note that the index-value pairs in a
mapping are not stored in a specific order. You can not refer to the
fourteenth key-index pair, since there is no way of telling which one is
the fourteenth. Because of this, you cannot use the range operator on
mappings.
The following operators and functions are important:
- indexing ( m [ ind ] )
- As discussed above, indexing is used to retrieve, store and add values
to the mapping.
- addition, subtraction, union, intersection and xor
- All these operators works exactly as on arrays, with the difference that
they operate on the indices. In those cases when the value can come from
either mapping, it will be taken from the right side of the operator.
This makes it easier to add new values to a mapping with +=.
Some examples:
([1:3, 3:1]) + ([2:5, 3:7]) returns ([1:3, 2:5, 3:7 ])
([1:3, 3:1]) - ([2:5, 3:7]) returns ([1:3])
([1:3, 3:1]) | ([2:5, 3:7]) returns ([1:3, 2:5, 3:7 ])
([1:3, 3:1]) & ([2:5, 3:7]) returns ([3:7])
([1:3, 3:1]) ^ ([2:5, 3:7]) returns ([1:3, 2:5])
- same ( a == b )
- Returns 1 if a is the same mapping as b, 0 otherwise.
- not same ( a != b )
- Returns 0 if a is the same mapping as b, 1 otherwise.
- array indices(mapping m)
- Indices returns an array containing all the indices in the mapping m.
- mixed m_delete(mapping m, mixed ind)
- This function removes the index-value pair with the index ind from the mapping m.
It will return the value that was removed.
- int mappingp(mixed m)
- This function returns 1 if m is a mapping, 0 otherwise.
- mapping mkmapping(array ind, array val)
- This function constructs a mapping from the two arrays ind and
val. Element 0 in ind and element 0 in val becomes
one index-value pair. Element 1 in ind and element 1 in val
becomes another index-value pair, and so on..
- mapping replace(mapping m, mixed from, mixed to)
- This function creates a copy of the mapping m with all values equal to from replaced by to.
- mixed search(mapping m, mixed val)
- This function returns the index of the 'first' index-value pair which has the value val.
- int sizeof(mapping m)
- Sizeof returns how many index-value pairs there are in the mapping.
- array values(mapping m)
- This function does the same as indices, but returns an array with all the values instead. If indices and values are called on the same mapping after each other, without any other mapping operations in between, the returned arrays will be in the same order. They can in turn be used as arguments to mkmapping to rebuild the mapping m again.
- int zero_type(mixed t)
- When indexing a mapping and the index is not found, zero is returned. However, problems can arise if you have also stored zeroes in the mapping. This function allows you to see the difference between the two cases. If zero_type(m [ ind ]) returns 1, it means that the value was not present
in the mapping. If the value was present in the mapping, zero_type will return something else than 1.