4.2.5 object
Although programs are absolutely necessary for any application you might
want to write, they are not enough. A program doesn't have anywhere
to store data, it just merely outlines how to store data. To actually store
the data you need an object. Objects are basically a chunk of memory
with a reference to the program from which it was cloned. Many objects can
be made from one program. The program outlines where in the object
different variables are stored.
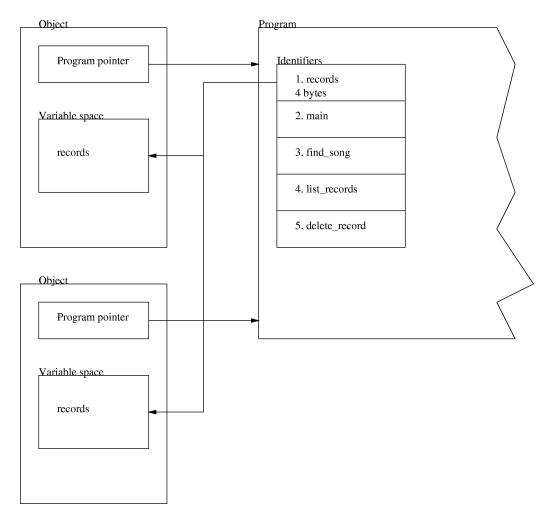
fig 4.5
Each object has its own set of variables, and when calling a function in that
object, that function will operate on those variables. If we take a look at
the short example in the section about programs, we see that it would be
better to write it like this:
class record {
string title;
string artist;
array(string) songs;
void show()
{
write("Record name: "+title+"\n");
write("Artist: "+artist+"\n");
write("Songs:\n");
foreach(songs, string song)
write(" "+song+"\n");
}
}
array(record) records = ({});
void add_empty_record()
{
records+=({ record() });
}
void show_record(object rec)
{
rec->show();
}
Here we can clearly see how the function show prints the
contents of the variables in that object. In essence, instead of accessing
the data in the object with the -> operator, we call a function
in the object and have it write the information itself. This type of
programming is very flexible, since we can later change how record
stores its data, but we do not have to change anything outside of
the record program.
Functions and operators relevant to objects:
- indexing
- Objects can be indexed on strings to access identifiers. If the identifier
is a variable, the value can also be set using indexing. If the identifier
is a function, a pointer to that function will be returned. If the
identifier is a constant, the value of that constant will be returned.
Note that the -> operator is actually the same as indexing.
This means that o->foo is the same as o["foo"]
- cloning
- As discussed in the section about programs, cloning a program can be done
in two different ways:
Whenever you clone an object, all the global variables will be
initialized. After that the function create will be called
with any arguments you call the program with.
- void destruct(object o)
- This function invalidates all references to the object o and
frees all variables in that object. This function is also called when
o runs out of references. If there is a function named
destroy in the object, it will be called before the actual
destruction of the object.
- array(string) indices(object o)
- This function returns a list of all identifiers in the object o.
- program object_program(object o)
- This function returns the program from which o was cloned.
- int objectp(mixed o)
- This function returns 1 if o is an object, 0 otherwise.
Note that if o has been destructed, this function will return 0.
- object this_object()
- This function returns the object in which the interpreter is currently
executing.
- array values(object o)
- This function returns the same as rows(o,indices(o)).
That means it returns all the values of the identifiers in the
object o.
- comparing
- As with all data types == and != can be used to
check if two objects are the same or not.