4.2.1 array
Arrays are the simplest of the pointer types. An array is merely a block of
memory with a fixed size containing a number of slots which can hold any
type of value. These slots are called elements and are accessible
through the index operator. To write a constant array you enclose the
values you want in the array with ({ }) like this:
({ }) // Empty array
({ 1 }) // Array containing one element of type int
({ "" }) // Array containing a string
({ "", 1, 3.0 }) // Array of three elements, each of different type
As you can see, each element in the array can contain any type of value.
Indexing and ranges on arrays works just like on strings, except with
arrays you can change values inside the array with the index operator.
However, there is no way to change the size of the array, so if you want
to append values to the end you still have to add it to another array
which creates a new array. Figure 4.1 shows how the schematics of an array.
As you can see, it is a very simple memory structure.
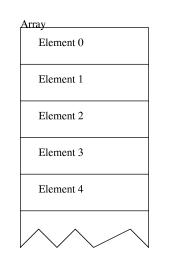
fig 4.1
Operators and functions usable with arrays:
- indexing ( arr [ c ] )
- Indexing an array retrieves or sets a given element in the array.
The index c has to be an integer. To set an index, simply put
the whole thing on the left side of an assignment, like this:
arr [ c ] = new_value
- range ( arr [ from .. to ] )
- The range copies the elements from, from+1, , from+2 ... to into a new array. The new array will have the size to-from+1.
- comparing (a == b and a != b)
- The equal operator returns 1 if a and b are the same arrays. It is not enough that they have the same size and same data. They must
be the same array. For example: ({1}) == ({1}) would return 0, while
array(int) a=({1}); return a==a; would return 1. Note that you cannot
use the operators >, >=, < or <= on arrays.
- Summation (a + b)
- As with strings, summation concatenates arrays. ({1})+({2}) returns ({1,2}).
- Subtractions (a - b)
- Subtracting one array from another returns a copy of
a with all the elements that are also present in b removed.
So ({1,3,8,3,2}) - ({3,1}) returns ({8,2}).
- Intersection (a & b)
- Intersection returns an array with all values that are present in both
a and b. The order of the elements will be the same as
the the order of the elements in a. Example:
({1,3,7,9,11,12}) & ({4,11,8,9,1}) will return:
({1,9,11}).
- Union (a | b)
- Union works almost as summation, but it only adds elements not
already present in a. So, ({1,2,3}) | ({1,3,5}) will
return ({1,2,3,5}).
Note: the order of the elements in a can be changed!
- Xor (a ^ b)
- This is also called symmetric difference. It returns an array with all
elements present in a or b but the element must NOT
be present in both. Example: ({1,3,5,6}) ^ ({4,5,6,7}) will
return ({1,3,4,7}).
- Division (a / b)
- This will split the array a into an array of arrays. If b is
another array, a will be split at each occurance of that array.
If b is an integer or float, a will be split between
every bth element. Examples: ({1,2,3,4,5})/({2,3}) will
return ({ ({1}), ({4,5}) }) and ({1,2,3,4})/2 will
return ({ ({1,2}), ({3,4}) }).
- Modulo (a % b)
- This operation is valid only if b is an integer. It will return
the part of the array that was not included by dividing a by
b.
- array aggregate(mixed ... elems)
- This function does the same as the ({ }) operator; it creates an
array from all arguments given to it. In fact, writing ({1,2,3})
is the same as writing aggregate(1,2,3).
- array allocate(int size)
- This function allocates a new array of size size. All the elements
in the new array will be zeroes.
- int arrayp(mixed a)
- This function returns 1 if a is an array, 0 otherwise.
- array column(array(mixed) a, mixed ind)
- This function goes through the array a and indexes every element
in it on ind and builds an array of the results. So if you have
an array a in which each element is a also an array. This function
will take a cross section, by picking out element ind from each
of the arrays in a. Example:
column( ({ ({1,2,3}), ({4,5,6}), ({7,8,9}) }), 2) will return
({3,6,9}).
- int equal(mixed a, mixed b)
- This function returns 1 if if a and b look the same. They
do not have to be pointers to the same array, as long as they are the same
size and contain equal data.
- array Array.filter(array a, mixed func, mixed ... args)
- filter returns every element in a for which func returns true when called with that element as first argument, and args for the second, third, etc. arguments.
- array Array.map(array a, mixed func, mixed ... args)
- This function works similar to Array.filter but returns the results
of the function func instead of returning the elements from a for which func returns true.
- array replace(array a, mixed from, mixed to)
- This function will create a copy of a with all elements equal to
from replaced by to.
- array reverse(array a)
- Reverse will create a copy of a with the last element first, the last but one second, and so on.
- array rows(array a, array indexes)
- This function is similar to column. It indexes a with
each element from indexes and returns the results in an array.
For example: rows( ({"a","b","c"}), ({ 2,1,2,0}) ) will return
({"c","b","c","a"}).
- int search(array haystack, mixed needle)
- This function returns the index of the first occurrence of an element
equal (tested with ==) to needle in the array
haystack.
- int sizeof(mixed arr)
- This function returns the number of elements in the array arr.
- array sort(array arr, array ... rest)
- This function sorts arr in smaller-to-larger order. Numbers, floats
and strings can be sorted. If there are any additional arguments, they
will be permutated in the same manner as arr. See
chapter 16 "Builtin functions" for more details.
- array uniq(array a)
- This function returns a copy of the array a with all duplicate
elements removed. Note that this function can return the elements
in any order.