6.5 Inherit
A big part of writing object oriented code is the ability to add functionality
to a program without changing (or even understanding) the original code.
This is what inherit is all about.
Let's say I want to change the hello_world program to write a version number
before it writes hello world, using inherit I could do this like
this:
inherit "hello_world";
int main(int argc, array(string) argv)
{
write("Hello world version 1.0\n");
return ::main(argc,argv);
}
What inherit does is that it copies all the variables and functions from the
inherited program into the current one. You can then re-define any function
or variable you want, and you can call the original one by using a ::
in front of the function name. The argument to inherit can be one of the following:
- A string
- This will have the same effect as casting the string to a program and then
doing inherit on this program.
- A constant containing a program.
- Any constant from this program, module or inherited program that contains a
program can be inherited.
- A class name
- A class defined with the class keyword is in fact added as a constant,
so the same rule as above applies.
Let's look at an example. We'll split up an earlier example into three parts
and let each inherit the previous part. It would look something like this:
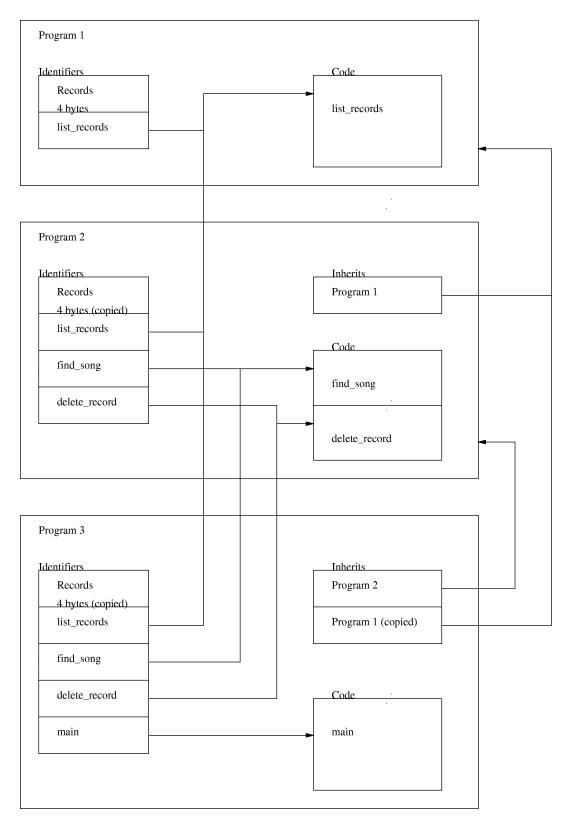
Note that the actual code is not copied, only the list of references.
Also note that the list of inherits is copied when you inherit a program.
This does not mean you can access those copied inherits with the ::
operator, it is merely an implementation detail. Although this example does
not show an example of a re-defined function, it should be easy to see how
that works by just changing what an identifier is pointing at.